What are Events?
All actions on a computer, such as clicking a button or opening a file, are considered events. Events allow the developer to create a non-blocking, asynchronous flow of control in their application.
When a script needs to fetch data from a database, it will send the request and continue execution without waiting for a reply. When the data is returned, an event is triggered, and a callback function is executed.
This allows the script to continue executing other tasks while it is waiting for the data to be returned, rather than being blocked. With this, the application is no longer reliant on a traditional blocking, synchronous flow of control.
In Node.js, objects have the ability to trigger events. There are several built-in objects that can trigger events. The most common of such built-in objects include:
- EventEmitter: The EventEmitter class allows developers to create custom events and register listeners for those events. This is the base class for many other objects in Node.js.
- Stream: The base of the HTTP request and response objects, the Stream class can trigger events when data is received, when a connection is established, and when there is an error
- Timers: The
setTimeout()
andsetInterval()
functions in Node.js are built on top of the Timers module, which allows the triggering events at specific time intervals.
- Child Process: This allows for the creation and management of child processes, a process created by another process to manage subtasks. The Child Process object can trigger events when the child process sends data, sends a message, disconnects, or exits.
- File system: The
fs
module in Node.js can trigger events when a file is opened, closed, or when data is read from a file.
Event Emitter
The Events module in Node.js is a built-in module that allows developers to create, fire and listen for their own custom events. It provides an event emitter class that can be used to create objects that can emit events and register listeners for those events.
To include built-in Events module in our project, we can use the
require()
method. The module provides an EventEmitter class. To be able to access these properties and methods we’ll need for managing events, we then need to create an instance of the EventEmitter object. 
This instance of the EventEmitter object can be used to create, emit, and listen for custom events.
The most vital methods to the Event Emitter object are the "emit" method, which is used to fire an event, and the "on" method, which is used to register a listener. The “once” method is notable as well, used to register one-time listeners.
Event Listeners
The ‘on’ method is used to register a listener for a specific event. When an event is triggered by the
emit()
method, the function called by ‘on’ is executed.The basic syntax for the ‘on’ method involves calling the
on()
method through EventEmitter, then entering the name of the event and the function we want to call when an event is triggered:
We can create a function to handle the event and call it within the
on()
method. In the following example, we create a function manageEvents
which logs ‘eventName triggered!’ to the console each time ‘eventName’ is triggered:
If additional arguments are entered into the
emit()
method, we may pass them to the event manager. This is achieved with the syntax:eventEmitter.on(‘eventName’, arg1, arg2 => { console.log(‘eventname triggered’, arg1, arg2); });
Keep in mind that any number of additional arguments may be added, the syntax shown above uses 2 for convenience. For example, below shows the code for an Event Listener that listens for the event ‘eventName’ and a number, then logs them via the
on()
method to the console: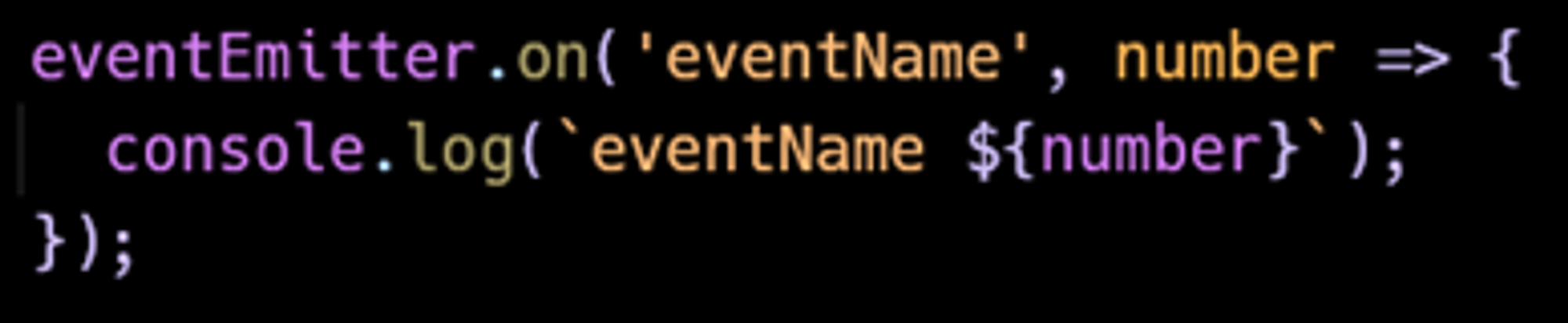
The
addListener()
method may also be used to register a listener function for a specific event. This is equivalent to the on()
method and fulfills the same purpose. The basic syntax is as follows:
For example, this listener registers a listener function for the "eventName" event, as the one we created with the ‘on’ method previously:

Additional methods that may be used on listeners include:
once()
: adds a one-time listener
removeListener()
/off()
: remove an event listener from the specified event
removeAllListeners()
: removes all listeners for an event
setMaxListeners()
: sets the maximum number of registrable listeners for an event
Firing events
The ‘on’ and ‘emit’ methods work in tandem when the ‘on’ method’s listener function for an event is triggered, the ‘emit’ method is the one triggering (emitting) the event. Thus to fire an event, use the
emit()
method.The basic syntax for the
emit()
method includes calling an instance of the Event Emitter class along with the name of the event. This is optionally followed by arguments that, when an event is emitted, are passed to the listener functions.
This example code fires the eventName event:

To demonstrate the interaction between the emit() and on() methods, this example code registers a listener function for the event ‘eventName’, which is triggered by the emit() method, and calls the function manageEvents to log the activity to console:
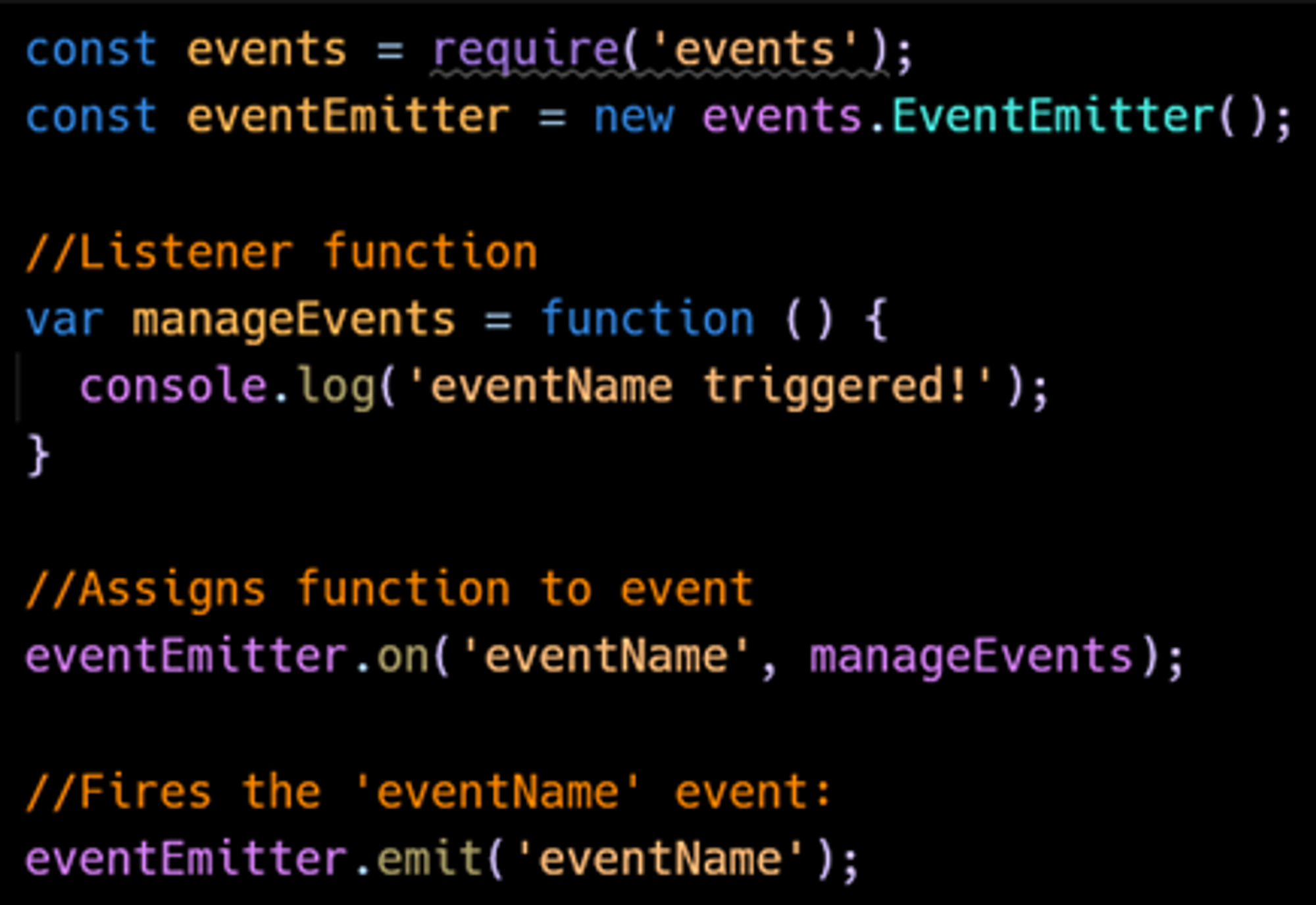
Methods
Below is a table of the most important methods in Event Emitter (several should be familiar from earlier in this chapter):
Method name | Function |
eventEmitter.emit(event [, arg1][, arg2][, ...]) | Triggers the events specified in its arguments. |
eventEmitter.on(event, listener) | Adds a listener for the specified event (allows duplicate listeners). Is identical to the addListener method. |
Adds a listener to the end of the listeners array for the specified event (allows duplicate listeners). | |
eventEmitter.once(event, listener) | Adds a one time listener for the event. This listener is invoked only the next time the event is fired, after which it is removed. |
eventEmitter.removeListener(event, listener) | Removes a listener for the specified event. Note: may change the order and indexes of the other listeners. |
eventEmitter.removeAllListeners([event]) | Removes all listeners, or those listening to the specified event. |
eventEmitter.setMaxListeners(n) | Sets maximum number of Event Listeners for each event to prevent memory leaks from failure to remove Event Listeners. By default, Node.js sets the maximum number of listeners to 10. |
eventEmitter.getMaxListeners() | Returns the current maximum listener value, either the default of 10 or the value set by the command above. |
eventEmitter.listeners(event) | Shows the list of listeners for the specified event. |
eventEmitter.listenerCount(type) | Returns the number of listeners for the type of event. |
Copyright © 2021 Code 4 Tomorrow. All rights reserved.
The code in this course is licensed under the MIT License.
If you would like to use content from any of our courses, you must obtain our explicit written permission and provide credit. Please contact classes@code4tomorrow.org for inquiries.