1.4 Printing to ConsoleWhat the Heck is the Console?Getting Started with ConsolePossibilities with Console.assert().log({}).table().time(__label__)Practice
1.4 Printing to Console
Console Meme
Me after writing Hello World with JavaScript:

What the Heck is the Console?
The console is the browser's built in debugger.
The console is your best friend for many reasons:
- It is faster and easier to use than other tools as well as being error free.
- It is a multi-purpose and somewhat generic tool.
- It is the most common debugging method used in JavaScript.
- It logs error messages, so you can easily debug them.
Getting Started with Console
To log (βsend message) to console, it is written as:
console.log("__insert_message_here__");
To find out all the possible options that
console
has to offer, it is written as:console.log(console);
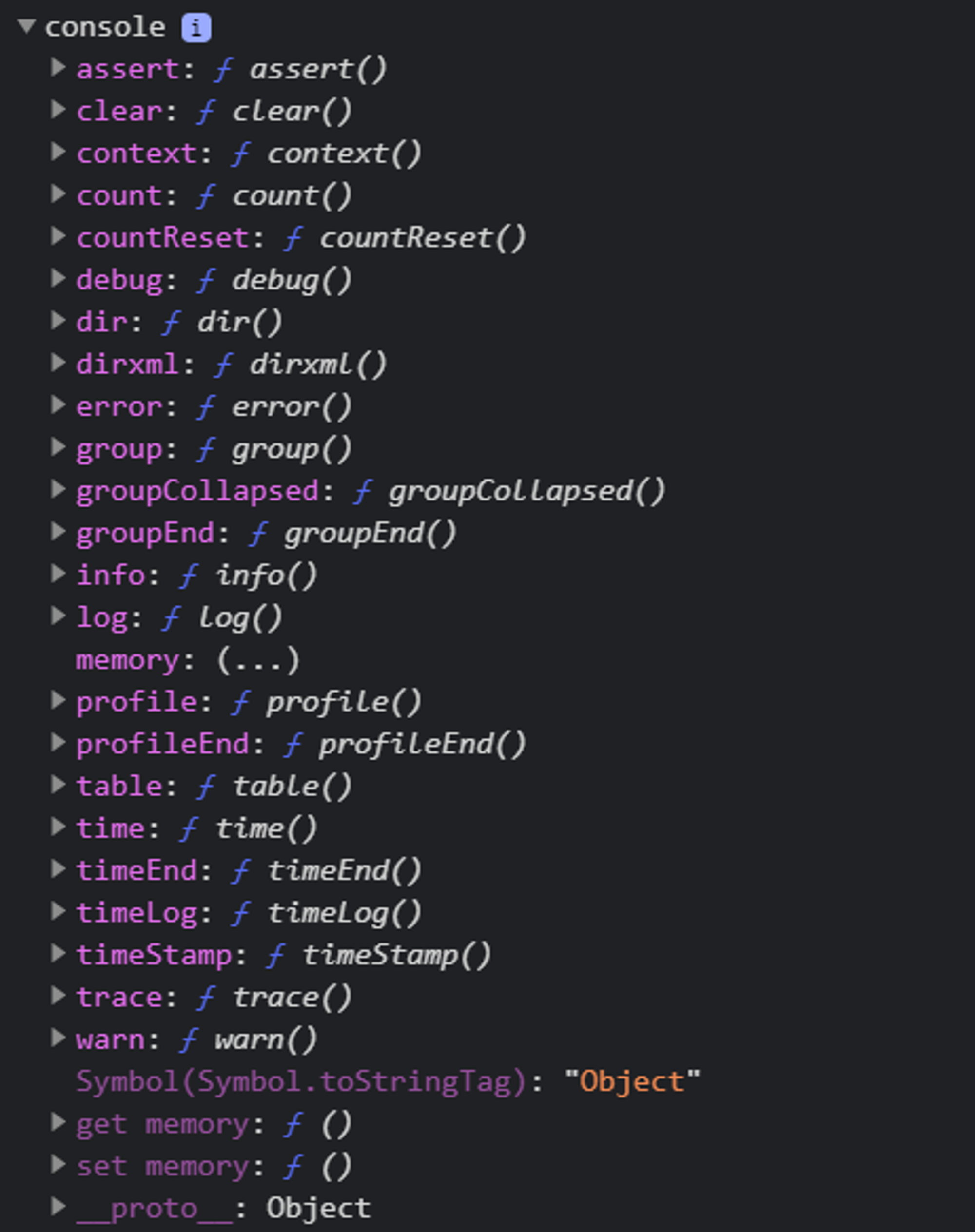
Possibilities with Console
.assert()
This section would make more sense after you learn conditional. So, if you don't get it right now, don't worry about it.
When you want to log something that is false, you use:
console.assert(__conditional__, 'user is not logged in');
So what is going on?
If some kind of conditional (β something that is defined as
true
or false
) is placed in place of __conditional__
and it has the value of false, then an error will pop up in the console, telling you the conditional is false..log({})
This provides labels for things that you desire to log.
// note: foo and bar are just variables let foo = 23; let bar = 24; console.log(foo, bar); // output: 23 24 console.log({foo, bar}); // output: { foo: 23, bar: 24 }
.table()
This provides you with a more organized output than
console.log({})
. let foo = 23; let bar = 24; console.table([foo, bar]);
The console should provide you with a table like this:

.time(__label__)
This starts a timer and
console.timeLog()
will log the time elapsed since that timer started.console.time('time'); console.timeLog('time');
Practice
You can add practice problems here if you want. Follow the format below.
π» Template code
Previous Section
1.3 Creating a JavaScript FileNext Section
1.5 Data TypesΒ
Copyright Β© 2021 Code 4 Tomorrow. All rights reserved.
The code in this course is licensed under the MIT License.
If you would like to use content from any of our courses, you must obtain our explicit written permission and provide credit. Please contact classes@code4tomorrow.org for inquiries.