Chapter Table of Contents
Ch. 2 OutputSection Table of Contents
Chapter Table of ContentsSection Table of ContentsWhat is a String?Why Variables?Declaration, Initialization, and Assignment of VariablesScope of VariablesNaming VariablesSummary: Do’s and Don’ts of Variable NamingConstantsUsing StringsHello World: A Closer LookPracticeVariable TypesApples and OrangesNext Section
What is a String?
What are strings? Well, you've actually been using them! A string is pretty much a collection of characters. We can think of them as sentences, words or phrases.
A string is also a type of variable. This is probably the first time you've heard of a variable. Imagine them as little packets of information.
Programming is all about storing, processing, and sharing data, whether it's between a user or to the printer. The way a computer remembers and keeps track of all these pieces of data is by storing them in variables.
If you wanted to store something, such as your name, in a variable, it would be of type
String
. This is where the syntax comes into play. In Java you have to tell the computer what sort of data the computer is going to store.Why Variables?
Watch this video for an introduction to what variables are and why they’re useful. You should stop watching at 0:32, since it gets into syntax at that point.
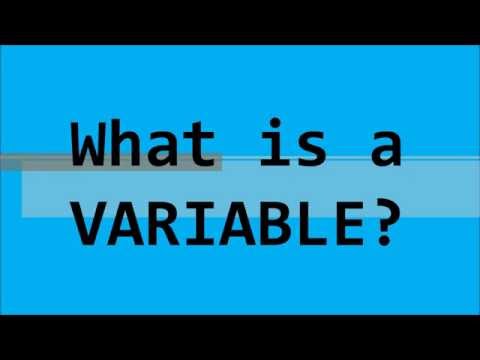
Declaration, Initialization, and Assignment of Variables
Before we dive into variables, let’s get some terms out of the way.
Declaration is when you tell the computer that the variable exists. In Java, you need to tell the computer what the type and name of the variable is. For example:
String name;
String
is the type of the variable, which goes before the name of the variable (which is name
).Initialization is when you give a declared variable a value for the first time. For example, the 2nd line initializes the variable
name
:String name; name = "Kaz"; //When initializing strings, you must use quotation-marks ""
Note that once a variable is declared, you cannot give it a value that doesn’t match the declared type. For example, you will later learn that there is a type called
int
which is for integers. You cannot do something like the code below because 5
is not a String.String name; name = 5; //The int variable type does not use quotation-marks
Note that when you are initializing a variable, you do not need to put the type again because the computer already knows what it is. In fact, if you try to declare a variable with the same name again, it will give you an error.
String name; int name; //Will give an error, make sure to name your variables unique names!
Another thing to note is that you can declare and initialize a variable in one step:
String name = "Kaz";
You can also declare or initialize multiple variables of the same type in one line, as long as you separate the declarations/initializations with commas:
// initialization and declaration in one line String firstName = "Kaz", lastName = "Brekker"; // declaration in one line String address, message;
To give the variable a new value once it has been initialized, you simply use the assignment operator
=
once again. You can assign new values to variables as many times as you want.String name = "Kaz"; // initialize name name = "Hello"; // assign name to a new value
Scope of Variables
All variables have a scope, or region of the code that it belongs to. Variables can only be accessed within their scope. You can see the scope of the variable by looking at where the variable is declared and the curly braces that enclose the variable. For example, the variable message belongs to the class A and the variable word belongs to class B:
class A { String message = "World"; } class B { String word = "java"; }
Naming Variables
Like naming classes, programmers have conventions for naming variables. Firstly though, you should read about variable naming rules so that your program doesn’t have any errors.
The generally accepted naming convention used in Java is called CamelCase. Basically, the first letter of each word in the variable is capitalized EXCEPT the first letter of the first word.
Here are some examples of good variable names:
firstName
phoneNumber
radius
isHungry
Here are some examples of bad variable names:
first_name
- This uses snake case, which is not used in Java except for constants (see below)S
PhoneNumber
- This makes it seem like PhoneNumber is a class
r
- This is just a letter and not descriptive enough
- (There are some exceptions to using letters as variable names, but generally speaking it’s better to be descriptive when naming variables)
Summary: Do’s and Don’ts of Variable Naming
Do
- Be descriptive, but concise
- Use CamelCase
Don't
- Use abbreviations or ambiguous shorthand
Constants
What if you want to initialize a variable whose value you never want to change, like the value of pi or a conversion rate? You should use constants. To declare a constant in Java, use the keyword
final
before the type of the variable:final int CENTS_PER_DOLLAR = 100;
The
final
keyword tells the computer that the variable should not be changed. If at any point your program attempts to assign a new value to a constant, the computer will give you an error.Notice that constants have different naming conventions from regular variables. Constants use snake case, where each word is separated by an underscore. Additionally, all of the letters in the name of the variable are uppercase.
Using Strings
So to use
String
, first you declare your variable to be of String
type. Notice that the ‘S’ in String
is capitalized. Then you give your variable a name of your choice. Next you assign the String
variable a value. Whatever text you type has to be in quotation marks, similar to what we did in System.out.println
.While printing it out to the console, you can use the addition symbol (
+
) to add two strings together. This is called concatenating.public class Name { public static void main(String[] args) { String firstName = "Jyoti"; String lastName = "Rani"; System.out.println(firstName + lastName); } }
What will the above code output?
Click arrow for answer
JyotiRani
Hello World: A Closer Look
Let's take a look again at the
HelloWorld
class from Setting Up Java. The System.out
portion emphasizes that the data is being printed as output to the console. Later on we will use System.in
for input (Chapter 5).Practice
Variable Types
Define different types of variables (see template code comments).
Apples and Oranges
- Define an integer variable called
numOranges
with value10
- Define an integer variable called
numApples
with value24
- Print out the number of oranges using variables, output:
"I have 10 oranges."
- Print out the number of apples using variables, output:
"I have 24 apples."
Next Section
2.2 Formatting TextCopyright © 2021 Code 4 Tomorrow. All rights reserved.
The code in this course is licensed under the MIT License.
If you would like to use content from any of our courses, you must obtain our explicit written permission and provide credit. Please contact classes@code4tomorrow.org for inquiries.