Chapter Table of Contents
Ch. 1 Intro to JavaSection Table of Contents
Chapter Table of ContentsSection Table of ContentsFirst Download the Java CompilerHello World, Making a Project and ClassCommentsDeclaring ClassesStyleIdent ProperlyPut Curly Brackets in the Right PlaceClass names should have the first letter of each word capitalizedAdd Comments to Explain What's HappeningPracticeHello WorldCommentsAbout MeNext Section
First Download the Java Compiler
Let's start off by downloading or opening a Java Compiler. One such compiler app is Eclipse and JDK so that your laptop can run Java is the first step. This should be done before class, and below is a quick video that shows you exactly how to do it. For a web-based compiler, we recommend replit.com

In the video, the teacher says to download Java SE 8, which should work (and you can scroll down the website linked above to download it (Click on JDK download). However, you can also download newer versions of Java. The latest as of May 2020 is Java SE 14.
Eclipse is an IDE (Integrated Development Environment). Basically, it's a program that allows programmers to write code. It has a lot of helpful features which might seem a bit overwhelming at first, but don't worry, it's actually a lot simpler to use than you might think (see next video). Also, if you have coded before and you have your own preferred IDE or text editor you like to use, feel free to use that for this course.
Replit is also an IDE. It requires no download and works entirely on your web browser! However, there are storage limits on replit and it can compile slower.
Hello World, Making a Project and Class
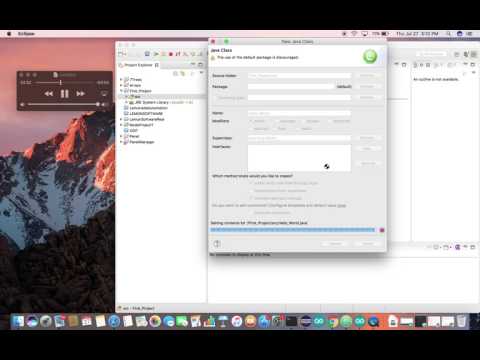
Note: Around 2:40-2:50, the teacher mentions that you should create a main class. It should actually be called the main method, not the main class. All of the code in the main method runs when you run your program.
Don't worry about understanding what all of the syntax means yet (such as
public
or class
). For now, just make sure you copy the code exactly as it appears.Comments
Do you see the
// TODO Auto-generated method stub
in green? That's an example of a single line Java comment. Comments are notes in our code that are ignored by the computer when the program runs. To form a single line comment, type in //
and then whatever message you want after that. To form a multi-line comment, start it with /*
and end it with */
. For example:// This is a single line comment /* This is a multi-line comment */
Usually, coders will put comments as a note to themselves or other people they're working with about how a certain snippet of code works. All good programs should have comments so that your code is readable by other human beings.
To un-comment something, simply remove the
//
or /*
and */
, and Java will consider that actual code.All Java statements end with a semicolon. An example of a statement is the
System.out.println
command.Declaring Classes
Classes are where you put the code for your program. In the video, the class was named
Hello_World
. To create a class, you simply write in the format:modifier(s) class ClassName { // class body }
For now, the only modifier you should have in front of the
class
keyword (a keyword is a word that has a special meaning in the programming language) is public
. In other words, you should declare your classes like so:public class ClassName { // class body }
We’ll learn more about modifiers later.
Style
What is good programming style? If you have good style, that means that you follow conventions agreed upon by all programmers when it comes to how you structure or write your programs.
Here are some conventions you should keep in mind from now on.
Ident Properly
Indent properly. Curly braces
{
and }
in Java represent the scope of certain structures, such as a class. What that means is that everything inside the {
and }
belongs to that class. Things that are inside the same set of curly braces should be indented on the same level. For example:public class Indent { public static void main(String[] args) { System.out.println("Hello"); System.out.println("World"); } }
The reason for this is to make clear the hierarchy of the code — the print statements above are part of the Indent class. This is an example of bad indentation:
public class Indent { public static void main(String[] args) { System.out.println("Hello"); System.out.println("World"); } }
As you can clearly see, this does not show that the print statements are part of the
main
method and are inconsistent.Put Curly Brackets in the Right Place
There are 2 accepted ways to place curly braces: end-of-line style or next-line style. Below are examples for both:
public class EndOfLine { // class body } public class NextLine { // class body }
Your IDE should automatically add an ending brace for you in the right place no matter which style you decide to work with.
Class names should have the first letter of each word capitalized
There should be no spaces, underscores, dashes, etc. between words. For example:
Good:
HelloWorld
MyJavaProgram
ClassName
Bad:
Hello-World
myjavaprogram
class_name
Add Comments to Explain What's Happening
While it’s true that a computer will be interpreting your code and running it, other human beings will look at your code too. That means you need to make your code readable, that is, you need to make it understandable to a human. Part of making code readable is adding comments to explain what’s happening in your program. For example:
// prints my age System.out.println(17);
However, what you don’t want to do is write redundant comments. For example, this is redundant:
// prints the number 17 System.out.println(17);
Basically, your comments should be concise but provide enough context for someone who has never seen your program before to understand what it does.
You should put comments above the line(s) of code that the comment refers to, or at the end of the line, like so:
System.out.println(17); // prints my age
Practice
Hello World
Create a new class called
HelloWorld
. In the main
method, print "Hello World!"
. Comments
Create a new class called
Comments
. In the main
method, write 1 single-line comment and 1 multi-line comment.About Me
Create a new class called
AboutMe
. In the main
method, print your name. On the same line, print any message. On the next line(s), print a stanza from your favorite poem. Make sure it's formatted correctly. And of course, be sure to have good programming style!Next Section
1.2 What is Java?Copyright © 2021 Code 4 Tomorrow. All rights reserved.
The code in this course is licensed under the MIT License.
If you would like to use content from any of our courses, you must obtain our explicit written permission and provide credit. Please contact classes@code4tomorrow.org for inquiries.