Break and Continue
When your program is running a loop, it has to finish the loop before it moves on to the next code. However, you can tell it to
break
out of the loop if you want it to. You can also tell the program to skip to the next iteration of the loop without completing the current iteration by using continue
.Break
For example, the below uses a break statement. It prompts the user to enter a number and while that number is less than 20, it will print all numbers from the given number up until 19. However, the
break
statement inside of the if
statement will make the while loop terminate if the current number is divisible by 4.x = int(input("Enter a number: ")) while x < 20: # break out of loop if # current is divisible by 4 if x % 4 == 0: break print(x) x += 1
Example output:
Enter a number: 13 13 14 15
As you can see, since 13 < 20, the while loop runs. 13, 14, and 15 will print because none of them are divisible by 4. However, once x is 16, the loop terminates because 16 is divisible by 4, which executes the
break
statement.Continue
On the other hand, the
continue
keyword will not terminate the loop; it will only terminate the current iteration of the loop. The code below uses a continue
statement. It is identical to the code above, except that it uses continue inside the if statement, and also increments x in the if statement (this is to avoid an infinite loop).x = int(input("Enter a number: ")) while x < 20: # don't print the number if it # is divisible by 4 if x % 4 == 0: x += 1 continue print(x) x += 1
Example output:
Enter a number: 13 13 14 15 17 18 19
Notice that this time, the loop runs for a longer time. Why? It's because continue doesn't terminate the loop, it just skips over the current iteration. 13, 14, and 15 aren't divisible by 4 and thus are printed. 16, however, is divisible by 4. Since the
continue
statement is executed this time, the program skips all the code after the continue
statement in the loop and starts anew. x is then 17, 18, 19, and 20. However, 20 is not printed because 20 < 20 is False, thus ending the while loop.Practice
Echo Enhanced
Write a program that continuously prompts the user to enter a message, and echoes that message back to the user (prints it). If the message is 'q', the program should end. If the message is 'c', the program shouldn't echo anything but instead prompt the user to enter another message to echo. See a demo of the program below
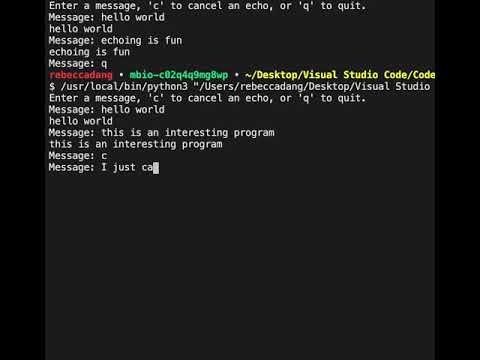
Copyright © 2021 Code 4 Tomorrow. All rights reserved.
The code in this course is licensed under the MIT License.
If you would like to use content from any of our courses, you must obtain our explicit written permission and provide credit. Please contact classes@code4tomorrow.org for inquiries.