Chapter Table of Contents
Ch. 9 ArraysSection Table of Contents
Chapter Table of ContentsSection Table of ContentsPracticeGrade EnhancementBilling EnhancementMad LibsAddress Book
Grade BookTelevision StatsVirus Stats
Practice
Grade Enhancement
Create a program called Grade which prompts the user for the number of test scores to be entered and then prompts the user to enter those scores. Then your program should print the letter grades that correspond to each score. (Use a
char[]
array to store the letter grades.)Sample output:
Enter the number of test score: 5 Enter score 1: 3 Enter score 1: 61 Enter score 1: 100 Enter score 1: 87 Enter score 1: 75 Grades: F D A B C
Billing Enhancement
Create a program called Billing which prompts the user for the number of items they will enter. Then ask the user for the name of the item and price repeatedly. Once the last item and its price has been entered, print a receipt which has each item and its price, as well as the subtotal, tax, and total. (The sales tax rate is 8%.) Hint: Use an array to store the item names and prices.
Sample output:
Enter the number of items: 3 Enter item #1: shirt enter the price of item #1: $15.43 Enter item #2: pants enter the price of item #2: $12.38 Enter item #3: hat enter the price of item #3: $4.99 RECEIPT ----------------------------------- shirt - $15.43 pants - $12.38 hat - $4.99 ----------------------------------- Subtotal: $32.80 Tax: $2.62 Total: $35.42
Hint: You may encounter a problem when using Scanner. Read the following article for a solution: Debugging Scanner Skipping Problem
Mad Libs
Create a program called MadLibs which prompts the person for different types of words (like in a game of Mad Libs) and then prints the Mad Libs story with the given words. You can use the Mad Libs template below, or you can search up your own!
Hint: Use an array to store the word types and the words themselves. You may also want to use String formatting to print the Mad Libs story.
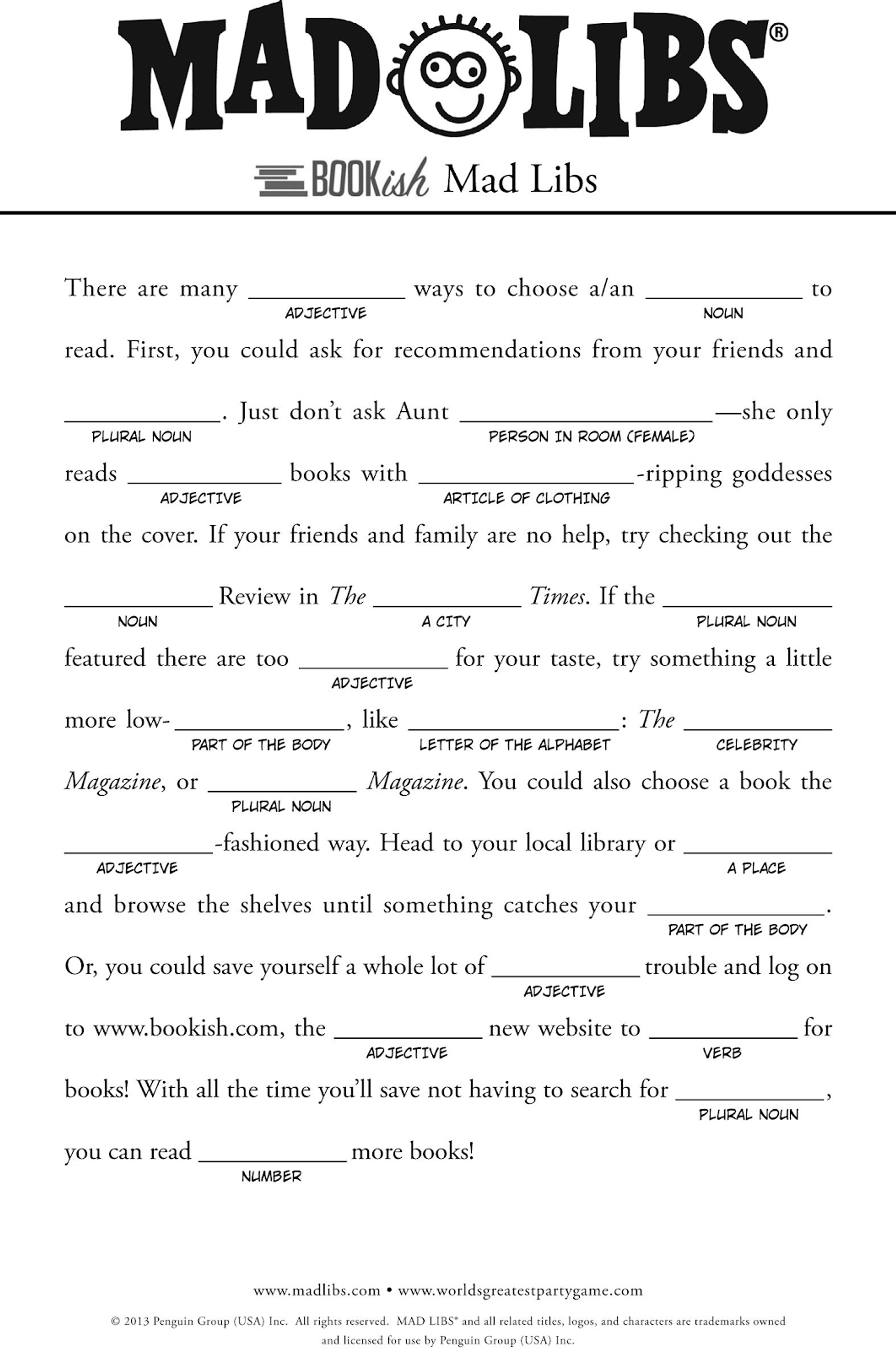
Sample output:
Enter a(n) adjective: old Enter a(n) noun: computer Enter a(n) plural noun: bottles Enter a(n) person in room (female): Rebecca Enter a(n) adjective: stinky Enter a(n) article of clothing: shirt Enter a(n) noun: doorman Enter a(n) city: San Jose Enter a(n) plural noun: pencil sharpeners Enter a(n) adjective: purple Enter a(n) part of the body: leg Enter a(n) letter of the alphabet: Q Enter a(n) celebrity: Ana de Armas Enter a(n) plural noun: apples Enter a(n) addjective: moldy Enter a(n) place: the pool Enter a(n) part of the body: finger Enter a(n) adjective: long Enter a(n) adjective: lanky Enter a(n) verb: glue Enter a(n) plural noun: keys Enter a(n) number: 1001 Bookish Mad Libs There are many old ways to chose a/an computer to read. First, you could ask for reccommendtations from your friends and bottles. Just don't ask Aunt Rebecca -- she only reads stinky books with shirt-ripping goddesses on the cover. If your friends and family are no help, try checking out the doorman Review in The San Jose Times. If the pencil sharpeners featured there are too purple for your taste, try something a little more low-leg, like Q: The Ana de Armes Magazine, or apples Magazine. You couold also choose a book the moldy-fashioned way. Head to your local library or the poola and browse the shelves until something catches your finer. Or, you could save yourself a whole lot of trouble and log on to www.bookish.com, the lanky new website to glue for books! With all the time you'll save not having to search for keys, you can readd 1001 more books!
Address Book
Create a program called AddressBook which prompts the user to enter 5 addresses. The program should prompt the user for the house number (
int
), street (String
), apartment (char
), and zip code (int
).Note that there is no
nextChar()
method in the Scanner class, so you will need to do something like this:Scanner input = new Scanner(System.in); char apt = input.nextLine().CharAt(0);
It should then print each of the addresses. If the user entered '-' for the apartment, the apartment should not be printed (see sample output).
The zip codes should always be printed so that they are 5 digits long. (You may need to use String formatting so that if the zip code is not 5 digits, it will fill in 0s to the left of the number until the entire zip code is 5 digits long. You can read about that here.)
Sample output: https://youtu.be/ms2tM-llTwo
Hint: You may encounter a problem when using Scanner. Read the following article for a solution: Debugging Scanner Skipping Problem
Grade Book
Create a program called GradeBook which prompts the user to enter information for 6 students. The program should ask the user for the student's first name (
String
), last initial (char
), student ID number (long
), points earned (int
), and points possible (int
). It should then print all of that data (see sample output).Sample output: https://youtu.be/2KC8Zy6EIwc
Hint: You may encounter a problem when using Scanner. Read the following article for a solution: Debugging Scanner Skipping Problem
Television Stats
Create a program called TelevisionStats which takes user input for a show and type of statistic and displays the statistic asked for. The program should end whenever the user enters "quit". Use separate 1D arrays for the type of stats, shows, seasons, episodes, wins, nominations, rating, start year, and end year.
The data you need can be found here.
Sample output: https://youtu.be/auKR4KX74q8
Virus Stats
Create a program called VirusStats which takes user input for a country and type of statistic and displays the statistic asked for. The program should end whenever the user enters "quit".
Use separate 1D arrays for the type of stats, countries, capitals, population, number of cases, deaths, recoveries, death rates, recovery rates, infection rates, and "risk grade." (Please note that "risk grades" are not based on actual scientific fact and were made up for the purposes of this exercise.)
The data you need can be found here.
To calculate the death rate: Take that country's deaths and divide it by the number of cases.
To calculate the recovery rate: Take that country's number of recoveries and divide it by the number of cases.
To calculate the infection rate: Take that country's number of cases and divide it by the population.
For the rates: Bonus points if you can round them (or format them) to the nearest hundredths place.
To determine the "risk grade", where 'A' is most dangerous and 'F' is least dangerous:
'A' if infection rate > 0.25 OR death rate > 25
'B' if infection rate > 0.2 OR death rate > 15
'C' if infection rate > 0.15 OR death rate > 10
'D' if infection rate > 0.1 OR death rate > 5
'F' for all else
Sample output: https://youtu.be/rFuGY2wXcKc
Copyright © 2021 Code 4 Tomorrow. All rights reserved.
The code in this course is licensed under the MIT License.
If you would like to use content from any of our courses, you must obtain our explicit written permission and provide credit. Please contact classes@code4tomorrow.org for inquiries.