Chapter Table of Contents
Ch. 6 ConditionalsSection Table of Contents
Conditionals are basically when the computer checks if a certain condition and runs a certain chunk of code depending on if it’s
true
or not. This is important for your coding because it gives you much more control since it represents a real life situation where different paths might be taken depending on different factors.The if statement
The simplest conditional is an if statement. The flow chart below summarizes how an if statement behaves in a program.
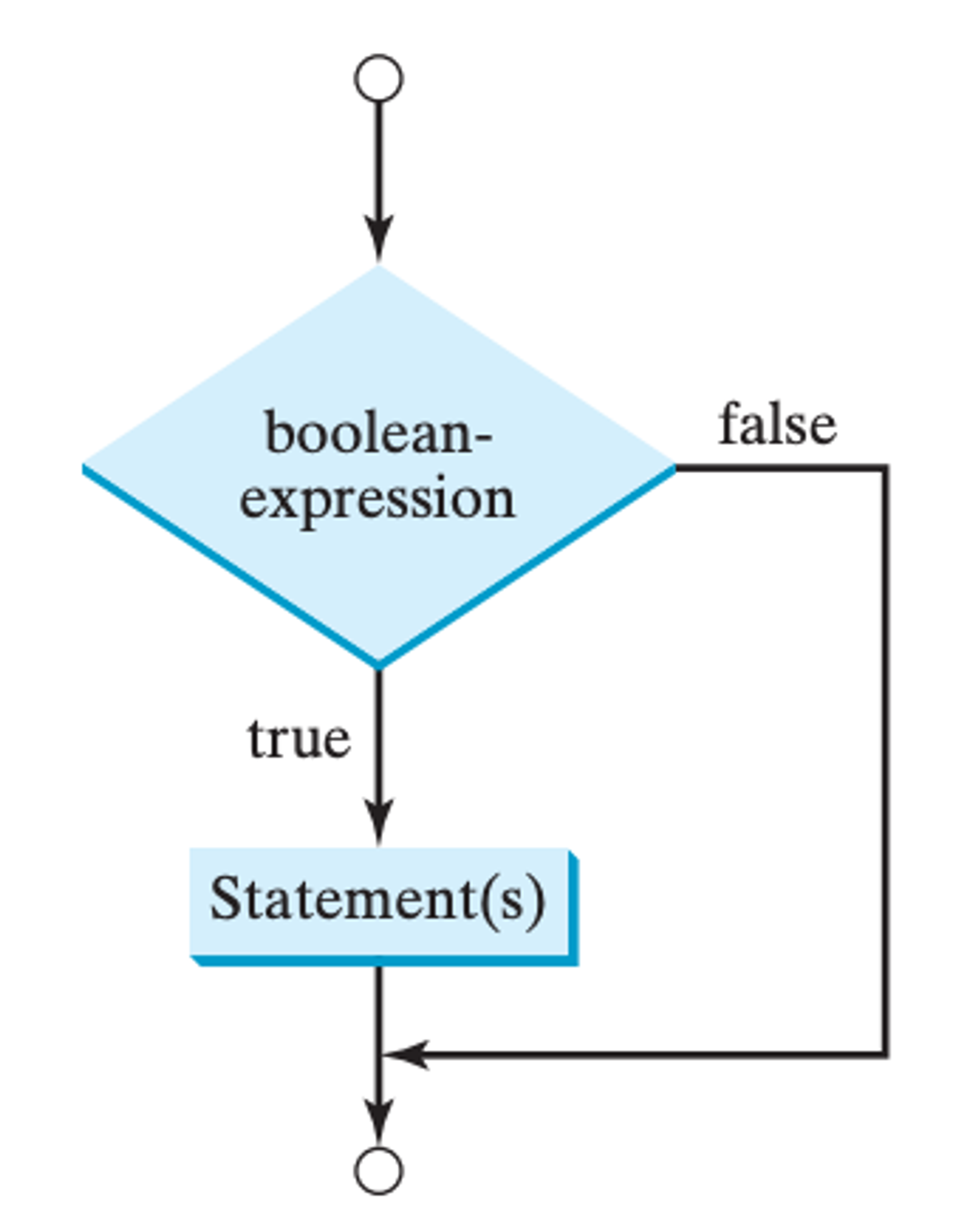
The code statements inside of the if block are only executed if a given condition is met. You actually use if statements in your daily life:
- If it is 7 am, then wake up
- If I am bored, then read a book
- If I have homework, then do homework
- If it is raining, then put on a raincoat
Here is the general structure of an if statement:
if (condition) { // if block code here }
Let’s take a look at an if statement in action. Analyze the code below. What do you think the program does?
// prompt user to enter their age Scanner input = new Scanner(System.in); System.out.print("Enter your age: "); int age = input.nextInt(); // you can vote if you're 18+ boolean canVote = age >= 18; if (canVote) { System.out.println("You can vote!"); }
In the program above, the user enters their age. If their age is 18+, then canVote is true and “You can vote!” is printed. Otherwise, nothing happens.
You might see why this is problematic — if we don’t print anything for someone who is, say, 5 years old, how will they know if they can vote or not? That’s where if-else statements come in.
The if-else statement
In an if-else statement, if the condition is
false
, the code inside of the else block runs. Analyze the code below. (Assume that the user is still prompted for their age, etc.)if (canVote) { System.out.println("You can vote!"); } else { System.out.println("Sorry, you can't vote yet."); }
In this case, the code does the same thing as the first program. However, we have the added benefit that if the person is younger than 18 years old, the program will print “Sorry, you can’t vote yet.”
Having if-else statements is nice, but what if I have more than 1 condition I want to check? That’s where else if comes in. You can use as many else if statements as you want to check multiple conditions. Analyze the code below.
if (canVote) { System.out.println("You can vote!"); } else if (age >= 16) { System.out.println("In California, you can register to vote."); } else { System.out.println("Sorry, you can't vote yet or register to vote in California."); }
Notice that the code in the else if block only runs if the previous condition was false and the current condition is true. In this case, if the user’s age isn’t 18+, the program will check if they are at least 16 years old. If they are, it will print: “In California, you can register to vote.” Otherwise, if it fails all of the conditions, it will print: “Sorry, you can’t vote yet or register to vote in California.”
Previous Section
6.3 Logical OperatorsNext Section
6.5 PracticeCopyright © 2021 Code 4 Tomorrow. All rights reserved.
The code in this course is licensed under the MIT License.
If you would like to use content from any of our courses, you must obtain our explicit written permission and provide credit. Please contact classes@code4tomorrow.org for inquiries.