Chapter Table of Contents
Ch. 3 DataSubsection Table of Contents
Word on Non-Primitives...
Non primitives such as
array
and String
are used in Java as objects. Objects can be identified because they are (almost always) created using the keyword new
. Objects, as you learned in the OOP lesson (see Ch. 1), are a key to Java and are powerful in the way they are manipulated. Objects are vital because they give you key and easy access to the data being manipulated.String
We've already done a bit with these, but now let's learn some handy methods that we will apply later. Manipulating strings is a big part of Java. Some examples are breaking up a string or setting all of the characters to lowercase or uppercase. Now why would this be important? Well it could be applicable in a user login system for example. So let's take a look at these methods.
// strings String username = "JYOTI"; String password = "rani"; System.out.println("Username: " + username); System.out.println("Password: " + password); System.out.println(username.charAT(3)); // string methods System.out.println(username.toLowerCase()); System.out.println(password.toUpperCase()); // strings are immutable - even after method calls, // username and password remain unchanged System.out.println("Username: " + username); System.out.println("Password: " + password);
Output of the code above:
Username: JYOTI; Password: rani; T jyoti RANI JYOTI Username: JYOTI Password: rani
In the above example, we use 4 new String methods. Methods are always followed by a set of parentheses, which is where the list of arguments (things that let you customize what a method does) goes. The 4 methods are:
toUpperCase()
returns a String where all of the characters are uppercase
toLowerCase()
- returns a String where all of the characters are lowercase
substring(start, end)
- returns a substring from the start index to the end index, exclusive (the character at the end index is not included)
charAt(index)
- returns the character at that index
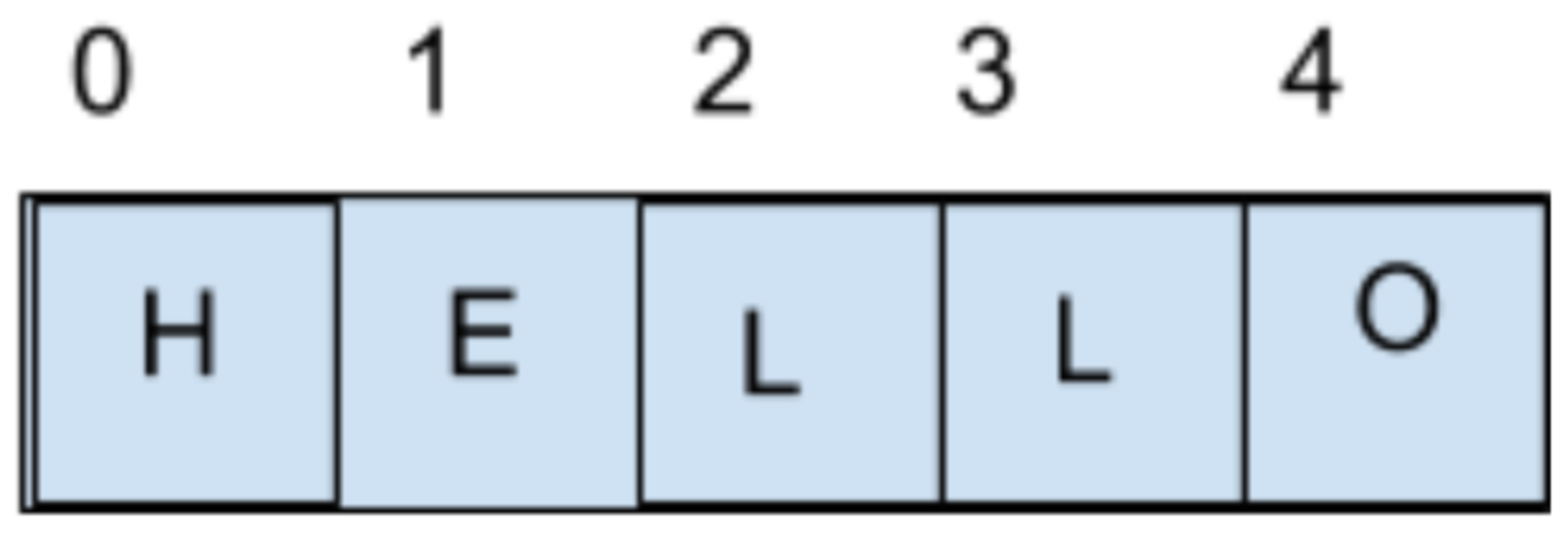
The picture represents how data in Strings are stored. Each character is at a certain position, or index, denoted by a number. Notice the indexing starts at 0, as with many aspects in Java. Usually, counting in the coding world starts with 0. With the word
“HELLO”
the substring specified at index from 0-4 would be “HELL”
because the substring method does not include the character at the ending index (index 4).We’ll learn more String methods in Ch. 10.
Note: Strings are immutable, which means that they cannot be changed. Even if it looks like a String is being “updated,” what is really happening behind the scenes is that the variable that holds the value of the String changes what location in memory it references.
For example, if a String
s
is initialized as "abcd"
, when you do s = "abcdef"
, you are not adding "ef"
to the first string. Rather, s just points to a different location in memory, as is demonstrated in the diagram below.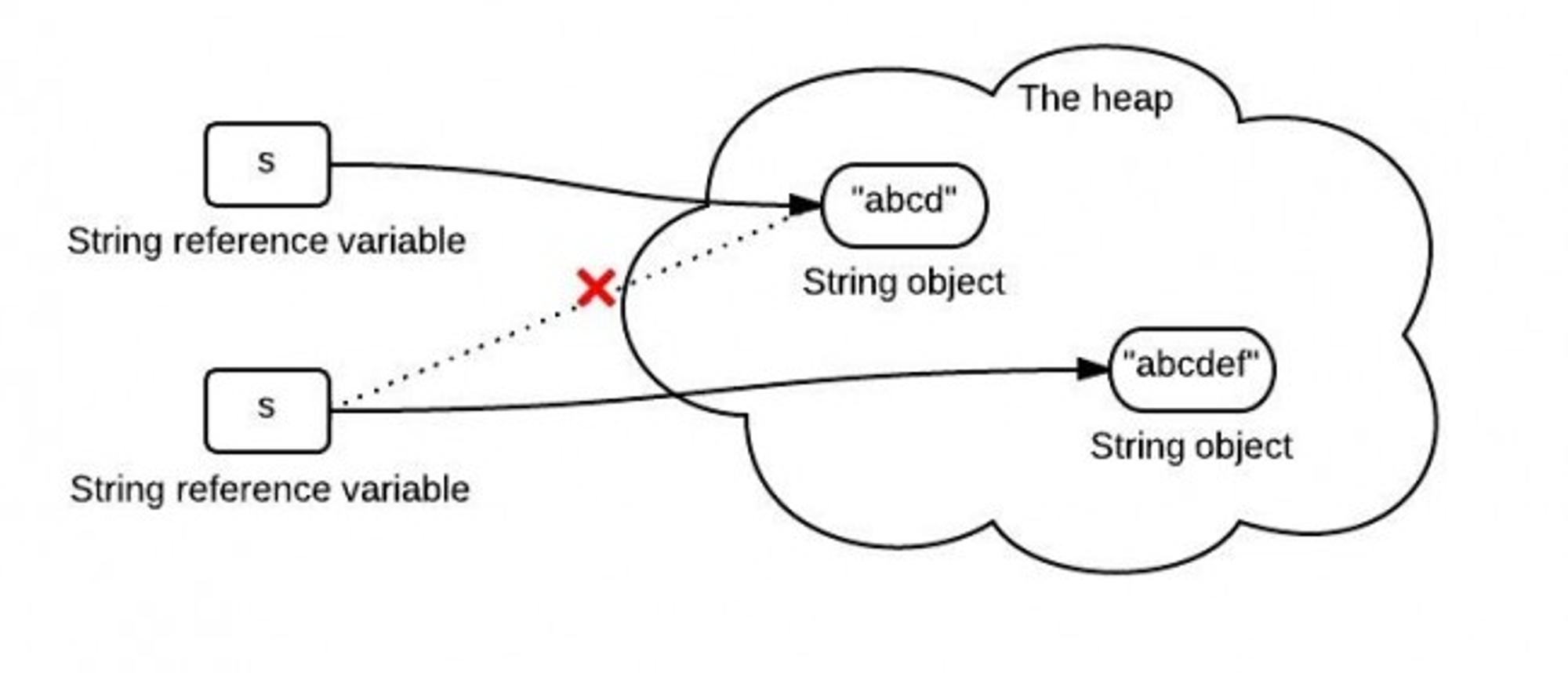
Array
Arrays are a non-primitive type as well. Arrays provide a way to organize data into an ordered list. You can have a 1D array (Ch. 9) or a multidimensional array, such as a 2D array (Ch. 10). Each part of an array is called an element. Similar to Strings, arrays are zero-indexed. We’ll get into arrays later, but for now you can look at this code as a preview for what arrays are like.
int{} numbers = {4, 5, 1, -1, 10, 6}; System.out.println("Element at index 2: " + numbers[2]);
Output of the code above:
Element at index 2: 1
Previous Section
3.1 Primitive TypesNext Section
3.3 Type-CastingCopyright © 2021 Code 4 Tomorrow. All rights reserved.
The code in this course is licensed under the MIT License.
If you would like to use content from any of our courses, you must obtain our explicit written permission and provide credit. Please contact classes@code4tomorrow.org for inquiries.