17.3 Stacks
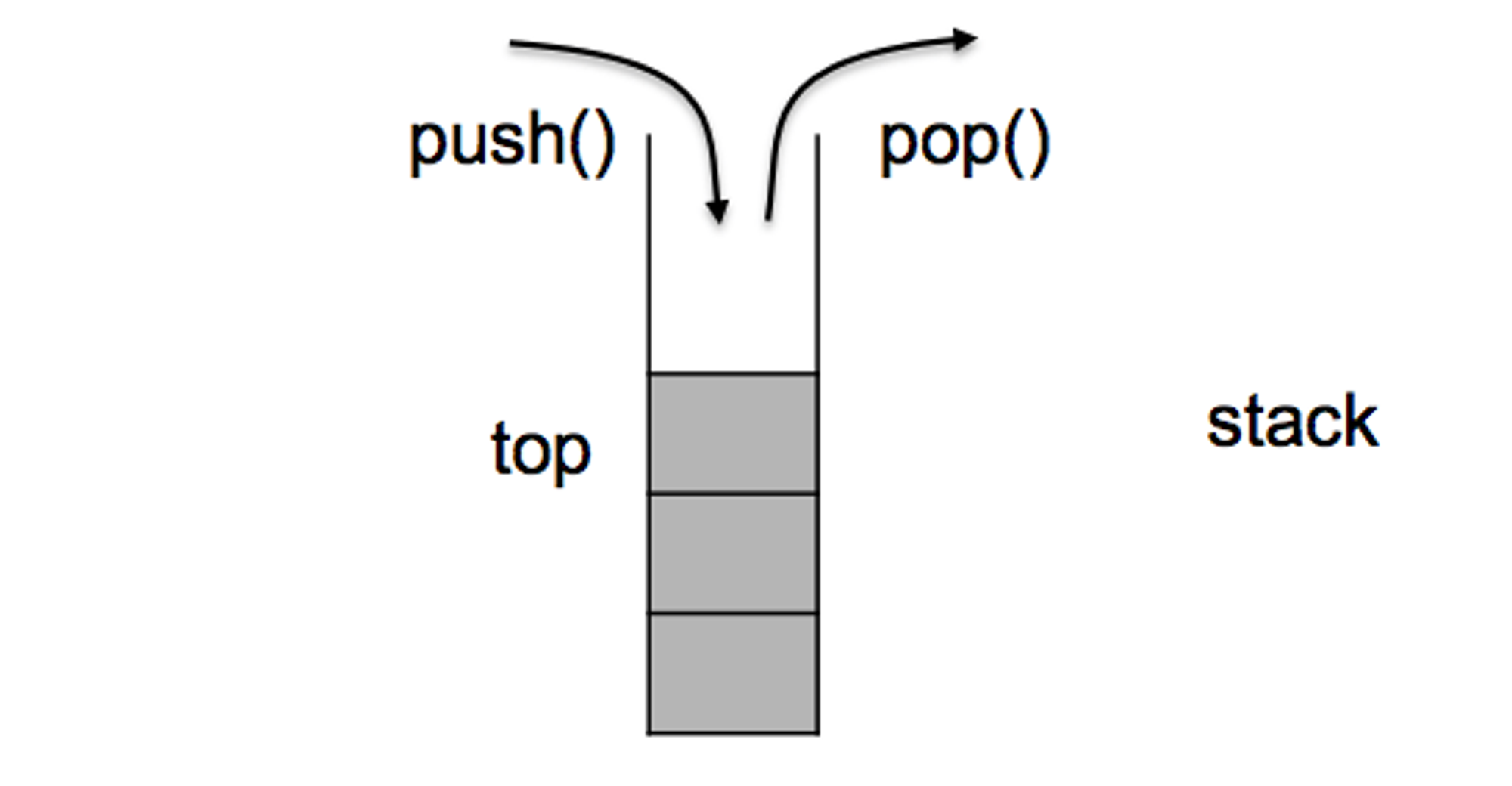
A stack is a similar structure to a Queue. However, it implements FILO instead of FIFO. In other words, instead of a line of elements, each element stacks on the last (looks like for once they got the naming right)!
The two main methods for stacks are
push()
and pop()
. These are easy to understand. The push method adds an element to the top of the stack, while the pop method removes the element from the top of the stack. Therefore, popping the top element would remove the last element pushed (think about it)! If you want to check the element at the top of the stack without removing it, use peek().Both Stacks and Queues extend the Vector class, which gives them abilities such as
indexOf()
and get()
.A non-comprehensive list of Stack methods is below from the documentation:

Note that the method returns a 1-based position. Make sure to remember! This is important because instead of the normal 0-indexed system the
search(Object)
method starts at 1. The indexes would be 1,2,3…. instead of 0,1,2….Yet again, we see the appearance of the Java Generic E. For now, you can think of E as a type of data in Java. You might have seen this before in
ArrayList<E>
. To help you understand this, some examples of E could be Integer, Double, even Character or String. This is where you see ArrayList<String>
or ArrayList<Integer>
. As a consequence, you use stacks by declaring them with the E. For example, a Stack of Integers would be:Stack<Integer> example = new Stack<Integer>();
However, note that it is possible to use it without the E, in which case you would just use:
Stack example = new Stack();
Lastly, note that the default constructor on a Stack initializes an empty stack. So if I were to run
example.empty()
, I would get true as a result.Previous Section
17.2 QueuesNext Section
Ch. 17 QuizCopyright © 2021 Code 4 Tomorrow. All rights reserved.
The code in this course is licensed under the MIT License.
If you would like to use content from any of our courses, you must obtain our explicit written permission and provide credit. Please contact classes@code4tomorrow.org for inquiries.
A stack is a similar structure to a Queue. However, it implements FILO instead of FIFO. In other words, instead of a line of elements, each element stacks on the last(looks like for once they got the naming right)!